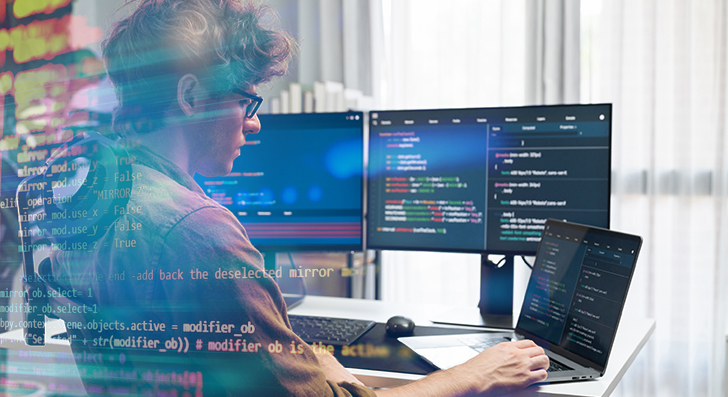
Scalability means your application can handle advancement—far more consumers, much more details, plus much more website traffic—with no breaking. Like a developer, building with scalability in your mind saves time and strain later on. Here’s a transparent and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't one thing you bolt on afterwards—it should be aspect of the plan from the start. Many apps fail every time they expand fast simply because the first design and style can’t manage the extra load. To be a developer, you must think early regarding how your method will behave under pressure.
Start out by developing your architecture to be versatile. Stay clear of monolithic codebases in which all the things is tightly linked. As a substitute, use modular layout or microservices. These styles break your app into scaled-down, independent areas. Each individual module or services can scale By itself devoid of impacting The complete system.
Also, think of your databases from working day one. Will it want to manage one million buyers or maybe 100? Pick the right kind—relational or NoSQL—determined by how your facts will mature. Plan for sharding, indexing, and backups early, even if you don’t require them still.
Another essential stage is to prevent hardcoding assumptions. Don’t compose code that only performs underneath present-day disorders. Consider what would transpire In the event your person foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use style patterns that assistance scaling, like message queues or celebration-pushed techniques. These aid your app deal with more requests without getting overloaded.
When you Establish with scalability in mind, you are not just planning for achievement—you are decreasing future problems. A very well-planned method is easier to maintain, adapt, and mature. It’s improved to get ready early than to rebuild later on.
Use the proper Databases
Picking out the proper database is often a key Component of constructing scalable applications. Not all databases are crafted the exact same, and using the wrong you can slow you down or simply induce failures as your application grows.
Start off by comprehending your details. Could it be highly structured, like rows in a very table? If yes, a relational database like PostgreSQL or MySQL is a good healthy. These are definitely sturdy with relationships, transactions, and regularity. They also guidance scaling strategies like browse replicas, indexing, and partitioning to manage more targeted traffic and information.
In case your facts is more versatile—like person action logs, products catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling substantial volumes of unstructured or semi-structured information and might scale horizontally more simply.
Also, consider your go through and produce patterns. Have you been accomplishing a lot of reads with fewer writes? Use caching and read replicas. Do you think you're managing a heavy compose load? Check into databases that can manage significant write throughput, and even function-primarily based knowledge storage devices like Apache Kafka (for non permanent data streams).
It’s also wise to Consider in advance. You might not have to have Sophisticated scaling functions now, but deciding on a databases that supports them means you won’t require to switch later.
Use indexing to speed up queries. Stay away from unneeded joins. Normalize or denormalize your knowledge determined by your obtain patterns. And usually keep track of database efficiency as you develop.
In brief, the correct database depends upon your app’s construction, pace demands, And the way you count on it to expand. Get time to pick wisely—it’ll help you save loads of hassle later on.
Enhance Code and Queries
Quickly code is vital to scalability. As your app grows, each small hold off provides up. Badly created code or unoptimized queries can decelerate general performance and overload your process. That’s why it’s crucial that you Construct productive logic from the start.
Start by crafting clear, straightforward code. Steer clear of repeating logic and take away everything needless. Don’t choose the most advanced Resolution if a simple one is effective. Maintain your functions small, targeted, and straightforward to check. Use profiling tools to search out bottlenecks—spots where your code usually takes way too prolonged to run or works by using a lot of memory.
Future, have a look at your database queries. These typically slow factors down more than the code by itself. Make sure Just about every query only asks for the info you actually have to have. Stay away from Pick out *, which fetches every little thing, and in its place choose precise fields. Use indexes to speed up lookups. And keep away from accomplishing too many joins, especially across substantial tables.
If you recognize a similar information currently being asked for again and again, use caching. Retailer the final results temporarily making use of instruments like Redis or Memcached so that you don’t really here need to repeat highly-priced operations.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your app extra efficient.
Remember to exam with large datasets. Code and queries that perform wonderful with a hundred documents could possibly crash when they have to handle 1 million.
In brief, scalable apps are quickly apps. Keep the code tight, your queries lean, and use caching when necessary. These measures support your software keep clean and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to deal with a lot more consumers and a lot more targeted traffic. If everything goes via 1 server, it'll rapidly become a bottleneck. That’s where load balancing and caching are available. Both of these instruments support maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic across numerous servers. Rather than one server doing many of the get the job done, the load balancer routes people to diverse servers depending on availability. This means no one server will get overloaded. If one particular server goes down, the load balancer can deliver visitors to the Other people. Tools like Nginx, HAProxy, or cloud-centered remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing facts briefly so it can be reused immediately. When end users request a similar data once more—like an item website page or possibly a profile—you don’t have to fetch it within the database every time. You may serve it within the cache.
There are 2 popular forms of caching:
1. Server-aspect caching (like Redis or Memcached) stores facts in memory for quick entry.
2. Shopper-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, improves pace, and makes your app a lot more economical.
Use caching for things that don’t improve usually. And normally ensure your cache is current when information does adjust.
In short, load balancing and caching are straightforward but impressive resources. Alongside one another, they help your app cope with far more buyers, stay rapidly, and Get better from problems. If you intend to increase, you would like each.
Use Cloud and Container Equipment
To develop scalable purposes, you'll need equipment that allow your application mature easily. That’s the place cloud platforms and containers can be found in. They offer you adaptability, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and services as you need them. You don’t need to acquire hardware or guess potential capability. When targeted visitors improves, you can add much more resources with just a few clicks or automatically utilizing auto-scaling. When traffic drops, you can scale down to save money.
These platforms also offer services like managed databases, storage, load balancing, and stability applications. You may center on building your application in place of controlling infrastructure.
Containers are Yet another important tool. A container offers your application and almost everything it has to operate—code, libraries, options—into 1 device. This can make it uncomplicated to move your app involving environments, from the laptop computer for the cloud, with out surprises. Docker is the preferred Resource for this.
When your application employs several containers, tools like Kubernetes make it easier to deal with them. Kubernetes handles deployment, scaling, and recovery. If just one element of your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate parts of your application into solutions. You could update or scale elements independently, which is perfect for overall performance and dependability.
In brief, working with cloud and container resources suggests you'll be able to scale speedy, deploy very easily, and Get better swiftly when complications occur. If you prefer your app to mature without having restrictions, begin working with these tools early. They preserve time, cut down danger, and allow you to continue to be focused on constructing, not correcting.
Monitor Almost everything
For those who don’t keep track of your application, you gained’t know when matters go Incorrect. Monitoring will help you see how your app is doing, location challenges early, and make much better choices as your app grows. It’s a critical Element of developing scalable techniques.
Start off by monitoring essential metrics like CPU use, memory, disk space, and response time. These let you know how your servers and companies are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you collect and visualize this information.
Don’t just watch your servers—observe your application much too. Regulate how much time it takes for users to load pages, how often errors happen, and where they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital complications. Such as, In the event your reaction time goes earlier mentioned a limit or possibly a provider goes down, you must get notified right away. This aids you repair issues fast, normally in advance of end users even recognize.
Monitoring is usually handy if you make adjustments. In the event you deploy a new element and see a spike in errors or slowdowns, you could roll it back again prior to it causes authentic injury.
As your app grows, traffic and facts boost. Without checking, you’ll skip indications of problems until it’s far too late. But with the correct applications in position, you stay in control.
In short, checking helps you maintain your application reputable and scalable. It’s not just about spotting failures—it’s about understanding your technique and making sure it works perfectly, even under pressure.
Remaining Feelings
Scalability isn’t only for huge companies. Even tiny applications require a robust Basis. By developing diligently, optimizing properly, and utilizing the right equipment, you could Develop applications that mature easily devoid of breaking under pressure. Commence smaller, Imagine large, and Make smart.